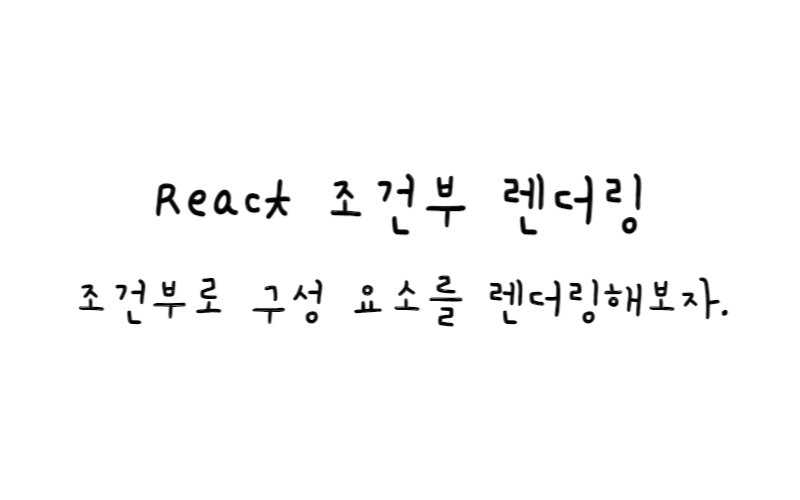
React
조건부 렌더링
React에서는 조건부로 구성 요소를 렌더링할 수 있다.
이를 수행하는 방법에는 여러 가지가 있다.
if 문
if 자바스크립트 연산자를 사용하여 렌더링할 구성 요소를 결정할 수 있습니다.
예제
두 가지 구성 요소를 사용할 것이다.
function MissedGoal() { return <h1>MISSED!</h1>; } function MadeGoal() { return <h1>Goal!</h1>; }
예제
이제 조건에 따라 렌더링할 구성 요소를 선택하는 다른 구성 요소를 생성한다.
function Goal(props) { const isGoal = props.isGoal; if (isGoal) { return <MadeGoal/>; } return <MissedGoal/>; } const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Goal isGoal={false} />);
기본 예시
예제 보기예제
isGoal 속성을 true로 변경한다.
const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Goal isGoal={true} />);
기본 예시
예제 보기논리 && 연산자
React 컴포넌트를 조건부로 렌더링하는 또 다른 방법은 && 연산자를 사용하는 것이다.
예제
중괄호를 사용하여 JSX에 자바스크립트 표현을 삽입할 수 있다.
function Garage(props) { const cars = props.cars; return ( <> <h1>Garage</h1> {cars.length > 0 && <h2> You have {cars.length} cars in your garage. </h2> } </> ); } const cars = ['Ford', 'BMW', 'Audi']; const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Garage cars={cars} />);
기본 예시
예제 보기
cars.length > 0이 true와 동일한 경우 && 뒤의 표현이 렌더링된다.
cars 배열을 비워보자.
예제
const cars = []; const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Garage cars={cars} />);
기본 예시
예제 보기삼항 연산자
요소를 조건부로 렌더링하는 또 다른 방법은 삼항 연산자를 사용하는 것이다.
예제
MadeGoal 구성 요소가 참이면 MadeGoal 구성 요소를 반환하고, 그렇지 않으면 MissedGoal 구성 요소를 반환한다.
function Goal(props) { const isGoal = props.isGoal; return ( <> { isGoal ? <MadeGoal/> : <MissedGoal/> } </> ); } const root = ReactDOM.createRoot(document.getElementById('root')); root.render(<Goal isGoal={false} />);
기본 예시
예제 보기참고
W3C School - React – React Conditional Rendering